Note: As you can see in the output, the positions of list items are changed. For strings, a list of characters is returned. Find centralized, trusted content and collaborate around the technologies you use most. Method # 1: Using List Comprehension + sample () + join () A combination of the above functions can be used to solve this problem. The sample() function returns a sample from a sequence as per the sample size you passed to it. How can the mass of an unstable composite particle become complex? punctuation ))) for word in words: if len ( word) < 4: continue mid = list ( word [ 1: -1 ]) random. For example, list1 = list([10, 20, 'a', 'b']), Use a random module to perform the random generations on a list, Use the random.shuffle(list1) function to shuffle a list1 in place.the shuffle() shuffles the original list, i.e., it changes the order of items in the original list randomly and doesnt return a new list. In this tutorial, we will look at how to scramble strings in a given Python list. They must be equal for s2 to be a scrambled version of s1. Wouldn't concatenating the result of two different hashing algorithms defeat all collisions? Dealing with hard questions during a software developer interview. Would the reflected sun's radiation melt ice in LEO? As shuffle() function doesnt return anything. rgeat / \ rg eat / \ / \ r g e at / \ a t We say that "rgeat" is a scrambled string of "great". When we create a string, this internally converts into a combination of 1s and 0s for the computer to handle. and Twitter for latest update. Follow me on Twitter. What do you think of this article on random.shuffle()? rgeat / \ rg eat / \ / \ r g e at / \ a t We say that "rgeat" is a scrambled string of "great". Loop through the first string and increment each character in the map by one. If you try to shuffle a generator object using it, you will get a TypeError: the object of type 'generator' has no len(). Because a string is an immutable type, and You cant modify the immutable objects in Python. Time Complexity: O(N^2), where N is the length of the given strings.Auxiliary Space: O(N^2), As we need to store O(N^2) string in our mp map. watercraft carrier boat string of words watercraft carrier boat string of words In theory this might work, but I'm not sure about re-runnning information through a logger that was otherwise saved in a log hander. Write a Python program to remove sublists from a given list of lists, which are outside a given range. Customize search results with 150 apps alongside web results. How to trim a string in Python Python has three built-in methods for trimming leading and trailing whitespace and characters from strings. The random.sample() function returns the random list with the sample size you passed to it. At what point of what we watch as the MCU movies the branching started? Previous: Write a Python program to remove sublists from a given list of lists, which are outside a given range. you can use more_itertools.random_permutation. The output string contains the letters of the word in a randomly shuffled manner. As you know, the shuffle() works in place and returns None, i.e., it changes the order of items in the original list randomly. Note that it shuffles in-place so you first have to convert your string to a list of characters, shuffle it, then join the result again. For example, str1 = "Hello, world!" str2 = "I love Python." Python strings are written between single quote marks like 'Hello' or alternately they can be written in double quote marks like "There". Python List Exercises, Practice and Solution: Write a Python program to scramble the letters of a string in a given list. We can keep the original list intact using the following two ways. Did you find this page helpful? PYnative.com is for Python lovers. For example, a schematic diagram of the indices of the string 'foobar' would look like this: String Indices. As the generator cannot provide us the size we need to convert it into a list to shuffle it. At the end, the value is removed from the temporary array. This function changes the position of items in a mutable sequence. That is, we do NOTwant the output to be of the form. A range() function returns a sequence of numbers. Unscramble the sentence. first install the python-string-utils library pip install python_string_utils use string_utils.shuffle () function to shuffle string please use the below snippet for it Code Snippet import string_utils print string_utils.shuffle ("random_string") Output: drorntmi_asng 2. Thus, ocred is a scrambled string of coder.Examples: Input: S1=coder, S2=ocderOutput: YesExplanation:ocder is a scrambled form of coderInput: S1=abcde, S2=caebdOutput: NoExplanation:caebd is not a scrambled form of abcde. How to rearrange letters from a word from a list that you have made? We doNOT want to shuffle the order of strings. Lets understand this with the help of the following example. Free coding exercises and quizzes cover Python basics, data structure, data analytics, and more. How do I split the definition of a long string over multiple lines? But most of the time, we need the original list or sequence. How do I replace all occurrences of a string in JavaScript? The steps involved are: There is a three-line version to this problem: import random as r a_string = "zbc" l = list (a_string) l [0] = "a" res = "".join (l) print (res) Output: abc Can I use a vintage derailleur adapter claw on a modern derailleur. How to Remove Leading and Trailing Whitespace from Strings in Python [python] >>> s = 'Don' >>> s 'Don' In fact, the name comes from the way in which the band moves between formations - members run to each form without using a predescribed path; this . How to shuffle the letters of string in python. How can I change a sentence based upon input to a command? So each time we run the code we shall get a different output. Lets assume if you want to Shuffle two lists and maintain the same shuffle order. To get New Python Tutorials, Exercises, and Quizzes. first_string = input ("Provide the first string: ") second_string = input ("Provide the second string: ") if sorted (first . Write a Python program to find the maximum and minimum values in a given heterogeneous list. Compare Two Strings We use the == operator to compare two strings. Required fields are marked *, By continuing to visit our website, you agree to the use of cookies as described in our Cookie Policy. These options dont modify the original list but return a new shuffled list. The different ways a word can be scrambled is called "permutations" of the word. Fetch all keys from a dictionary as a list. 2. When comparing values, Python always returns either "true" or "false" to indicate the result. Please note that the output changes each time as it is random. This work is licensed under a Creative Commons Attribution 4.0 International License. Here is a helper that uses random to non-destructively shuffle, string, list or tuple: To subscribe to this RSS feed, copy and paste this URL into your RSS reader. S2[0i] is a scrambled string of S1[0i] and S2[i+1n] is a scrambled string of S1[i+1n]. Basically, what it does is just take in a string, convert it to a list, and make a temporary copy. In this example, I am shuffling the list of Employee objects. Now that we have the list, we can convert it into a string using any of the methods givenhere. Also, try to solve the following exercise and quiz to have a better understanding of working with random data in Python. We can shuffle a string using various approaches. 3. How to Slice the String with Steps via Python Substrings You can slice the string with steps after indicating a start-index and stop-index. might become: 'elhloothtedrowl'. To make it work with any list, we need to find the exact seed root number for that list, which will produce the output we desire. The following code works in Google Colab. For instance I have a list of strings like: A list comprehension is an easy way to create a new list: From this you can build up a function to do what you want. Then shuffle the string contents and will print the string. The index of the last character will be the length of the string minus one. Use the join() method to concatenate to a single string again. To learn more, see our tips on writing great answers. According to our other word scramble maker, PYTHON can be scrambled in many ways. A-143, 9th Floor, Sovereign Corporate Tower, We use cookies to ensure you have the best browsing experience on our website. Python random.shuffle() function to shuffle list, Option 1: Make a Copy of the Original List, Option 2: Shuffle list not in Place using random.sample(), Shuffle Two Lists At Once With Same Order, Shuffle a List to Get the Same Result Every time, Shuffle a String by Converting it to a List, Approach Two: Shuffling a String, not in place, Shuffle list not in place to return a new shuffled list. Use the below steps to shuffle a list in Python, Create a list using a list() constructor. Each word is scrambled independently, and the results are concatenated. Are there any ways to scramble strings in python? Let us consider the word CodeSpeedy. This kind of problem can particularly occur in gaming domain. How do you scramble letters in a string in Python? I want to hear from you. Launching the CI/CD and R Collectives and community editing features for How to create a new string of a string with different combination, How to randomize every character from different user inputs. Lets see the example. Does With(NoLock) help with query performance? "Least Astonishment" and the Mutable Default Argument. It is pseudo-random (PRNG), i.e., deterministic. compile ( r'\s') words = splitter. The compiler will also give a string as output. I this instance, I would go for. These are taken from open source projects. This conversion of string to binary numbers is called encoding and is done using Unicode in Python. rev2023.3.1.43269. By voting up you can indicate which examples are most useful and appropriate. Pythons random module is not truly random. How can I recognize one? Little tips and tricks for working. What is the difficulty level of this exercise? Python (fun mini projects) Jumbling up a string in Python Andy Dolinski 4.79K subscribers Subscribe 59 Share 9.6K views 7 years ago Having fun with Python. To shuffle strings or tuples, use random.sample (), which creates a new object. ORMake a copy of the original list before shuffling (Ideal way). In this section, we will see how to shuffle String in Python. Make a copy of the original list before shuffling (Ideal and preferred), Use the random.sample() function to shuffle list not in place to get the new shuffled list in return instead of changing the original list. To shuffle numbers in the range(10), first convert the range to a list. Scramble Word (Python recipe) Scramble word re-arrange characters of word by using simple string manipulation and Random. You are given a scrambled input sentence. If the length of the string is > 1, do the following: Split the string into two non-empty substrings at a random index, i.e., if the string is s, divide it to x and y where s = x + y. import random import os from colorama import Style, Fore import colorama from click import pause import time colorama.init () def main (word): x = word l = list (x) random.shuffle (l) y = ''.join (l) print (Fore.YELLOW + ' [>] Output: ' + y + Style.RESET_ALL) #time.sleep (1) pause (Fore.RED + Style.BRIGHT + '\n [!] Why do I get "Pickle - EOFError: Ran out of input" reading an empty file? Like those before me, I'd use random.shuffle(): A little bit of styling added, but it does the job! 3. I don't know what translation was otherwise saved. You.com is an ad-free, private search engine that you control. You can shuffle a list in place with random.shuffle(). Using the word generator and word unscrambler for the letters P Y T H O N, we unscrambled the letters to create a list of all the words found in Scrabble, Words with Friends, and Text Twist. Remove empty strings from a list of strings. The python string decode () method decodes the string using the codec registered for its encoding. Using .format () function. The multiplication operator repeats our Python string the given number of times. ), Convert list and tuple to each other in Python, Convert a list of strings and a list of numbers to each other in Python, Cartesian product of lists in Python (itertools.product), Extract, replace, convert elements of a list in Python, Convert 1D array to 2D array in Python (numpy.ndarray, list), Unpack and pass list, tuple, dict to function arguments in Python, Extract and replace elements that meet the conditions of a list of strings in Python, Remove/extract duplicate elements from list in Python, Check if the list contains duplicate elements in Python, Initialize the random number generator with. It means shuffle a sequence x using a random function. They are as follows. We have a solution to this. Site design / logo 2023 Stack Exchange Inc; user contributions licensed under CC BY-SA. Why doesn't the federal government manage Sandia National Laboratories? random.sample() returns a list even when a string or tuple is specified to the first argument, so it is necessary to convert it to a string or tuple. Method #1 : Using list comprehension + sample () + join () The combination of above functions can be used to solve this problem. Am I being scammed after paying almost $10,000 to a tree company not being able to withdraw my profit without paying a fee. Remember, the results are different because we are randomly shuffling the word. Let,s see an example Example: print ('Hello PythonGuides!') Output will be Hello PythonGuides! Also, we dont need to convert the string to a list to get the shuffled string. According to Google, this is the definition of permutation: a way, especially one of several possible variations, in which a set or number of things can be ordered or arranged. Scramble String using Recursion Given a string s1, we may represent it as a binary tree by partitioning it to two non-empty substrings recursively. The random.shuffle() needs to know the sequences size to shuffle the sequence uniformly. Is lock-free synchronization always superior to synchronization using locks? I'm writing a program and I need to scramble the letters of strings from a list in python. 4. rgeat / \\ rg eat / \\ / \\r g e at / \\ a tWe say that rgeat is a scrambled string of great.Similarly, if we continue to swap the children of nodes eat and at, it produces a scrambled string rgtae. Dynamic Programming Solution: The above recursive Code can be optimized by storing Boolean values of substrings in an unordered map, so if the same substrings have to be checked again we can easily just get value from the map instead of performing function calls. Use the below steps to shuffle a list in Python Create a list Create a list using a list () constructor. We will look at a simple implementation of this task in Python. 2. from random import sample If S2 is a scrambled form of S1, then there must exist an index i such that at least one of the following conditions is true: Note: An optimization step to consider here is to check beforehand if the two strings are anagrams of each other. By using our site, you The code will take the string and convert that string to list. Create a map of characters and integers. join ( mid ), word [ -1 ]) w3resource. Strings in python are surrounded by either single quotation marks, or double quotation marks. Here, You can get Tutorials, Exercises, and Quizzes to practice and improve your Python skills. You can initialize a random number generator with random.seed(). The straightforward recursion will be very slow due to many repeated recursive function calls. Initialize the two string variables s1 and s2 of the same size. Strings and tuples are immutable, so random.shuffle() that modifies the original object raises an error TypeError. Flake8: Ignore specific warning for entire file, Python |How to copy data from one Excel sheet to another, Finding mean, median, mode in Python without libraries, Python add suffix / add prefix to strings in a list, Python -Move item to the end of the list, EN | ES | DE | FR | IT | RU | TR | PL | PT | JP | KR | CN | HI | NL, Python.Engineering is a participant in the Amazon Services LLC Associates Program, an affiliate advertising program designed to provide a means for sites to earn advertising fees by advertising and linking to amazon.com, Chat GPT: how neural networks will change our work and who will have to find a new one, Study: young Americans prefer iPhone because of iMessage, After the restrictions were imposed, Microsoft's Bing chatbot began to frequently say "let's move on to a new topic" in response. Python Programming Foundation -Self Paced Course, Python | Tokenizing strings in list of strings, Python | Remove empty strings from list of strings, Python - Find all the strings that are substrings to the given list of strings, Python | Convert list of tuples to list of strings, Python | Convert list of strings to list of tuples, Python program to convert a list of strings with a delimiter to a list of tuple, Python | Convert list of strings and characters to list of characters, Python | Filter a list based on the given list of strings, Python | Filter list of strings based on the substring list. Jordan's line about intimate parties in The Great Gatsby? Scramble String using RecursionGiven a string s1, we may represent it as a binary tree by partitioning it to two non-empty substrings recursively.Below is one possible representation of A = great: great / \\ gr eat / \\ / \\g r e at / \\ a tTo scramble the string, we may choose any non-leaf node and swap its two children.For example, if we choose the node gr and swap its two children, it produces a scrambled string rgeat. Using %s string. Your email address will not be published. Use print(list1) to display the original/resultant list. The random module uses the seed value as a base to generate a random number. How do you rearrange words in Python? We found a total of 36 words by unscrambling the letters in python. The only difference is that shuffle () is used to perform the scrambling task, rather than sample () is used. While using PYnative, you agree to have read and accepted our Terms Of Use, Cookie Policy, and Privacy Policy. How do I make the first letter of a string uppercase in JavaScript? Using this approach, we can keep the original string unchanged and get the new shuffled string in return. How do I read / convert an InputStream into a String in Java? Check if the first string is equal to the second string, return true. Of strings Exercises and Quizzes to Practice and Solution: write a Python to... In this section, we do NOTwant the output to be a scrambled version of.... Tips on writing great answers will print the string minus one a given list of,. Increment each character in the range to a list using a list in Python what do you think this. Shuffle a sequence of numbers total of 36 words by unscrambling the letters of strings three built-in methods trimming.: a little bit of styling added, but it does is just take in a given range am the! ( Python recipe ) scramble word ( Python recipe ) scramble word ( Python recipe ) scramble word re-arrange of! Results are different because we are randomly shuffling the word join ( mid ),,! Mass of an unstable composite particle become complex Employee objects, you agree to have better. This tutorial, we will look at how to shuffle two lists and maintain the same shuffle order in domain! ) method decodes the string contents and will print the string to tree... I.E., deterministic data structure, data analytics, and you cant modify the immutable objects Python... Are most useful and appropriate scammed after paying almost $ 10,000 to a single again. Compile ( r & # x27 ; t know what translation was otherwise saved the... Of list items are changed ) scramble word re-arrange characters of word by using our,. Scrambled version of s1 structure, data analytics, and the results are different because we are randomly the! Concatenating the result of two different hashing algorithms defeat all collisions parties in the great Gatsby with (. The sequences size to shuffle the sequence uniformly here, you agree to have read and accepted Terms. To solve the following example used to perform the scrambling task, rather than sample (:... ) method to concatenate to a command the methods givenhere CC BY-SA over multiple lines sequence using! Improve your Python skills we use cookies to ensure you have the best browsing experience on our.... Get `` Pickle - EOFError: Ran out of input '' reading an empty file, list! Before me, I am shuffling the list of lists, which are outside a heterogeneous. Start-Index and stop-index Python basics, data analytics, and Privacy Policy those before me, am... On writing great answers second string, convert it into a list in Python but most of original..., convert it into a string uppercase in JavaScript string unchanged and get new! To binary numbers is called & quot ; permutations & quot ; of how to scramble a string in python string using codec! Implementation of this task in Python you think of this article on random.shuffle ( ) is used the (! Search results with 150 apps alongside web results words = splitter the Default! Module uses the seed value as a list that you control to Slice the string to binary numbers is &! With random data in Python we have the best browsing experience on our website Ran out input! Output string contains the letters in Python are surrounded by either single quotation marks particularly occur in domain. A different output a list in place with random.shuffle ( ) constructor Stack Exchange Inc user! Sequence uniformly at what point of what we watch as the generator can provide. Characters is returned, use random.sample ( ), first convert the range to a single string again following ways... Same size same size the scrambling task, rather than sample ( ): a little bit of styling,... The order of strings a range ( ) make the first string is equal to the second,. Without paying a fee to generate a random function site design / logo 2023 Stack Exchange Inc user! Simple implementation of this article on random.shuffle ( ) constructor two strings we use the below steps to shuffle letters! Then shuffle the how to scramble a string in python uniformly the immutable objects in Python about intimate parties in the great Gatsby use (! Output, the results are concatenated immutable objects in Python with query performance indicate which examples are useful! Multiple lines by using simple string manipulation and random I change a based! Output, the value is removed from the temporary array we have the,. In JavaScript here, you the code we shall get a different output example, I shuffling. You agree to have read and accepted our Terms of use, Cookie,. Occurrences of a string, convert it into a string, this internally converts into combination. List using a list in Python 36 words by unscrambling the letters of the time, we need scramble! Strings, a list of lists, which are outside a given range be length! Seed value as a list in Python is, we will see how to the! String unchanged and get the shuffled string in Java, try to solve the following ways. Nolock ) help with query performance: & # x27 ; ) words =.... In gaming domain the end, the value is removed from the temporary array occurrences of a string return! 10,000 to a command length of the word in a string in Python examples! Want to shuffle a list using a random number found a total of 36 words by the. Given range uses the seed value as a list to get new Python Tutorials, Exercises, more... Task, rather than sample ( ), word [ -1 ] ).... Sublists from a given Python list during a software developer interview string to binary numbers is called encoding is! To scramble strings in Python letter of a string in Python following two ways random module uses seed... Time we run the code we shall get a different output NoLock ) help with query performance have made ways. Customize search results with 150 apps alongside web results sequences size to a! Improve your Python skills as per the sample size you passed to it internally! Modify the immutable objects in Python to Practice and Solution: write a Python program remove! For the computer to handle before me, I am shuffling the list, and Quizzes to and. Intact using the codec registered for its encoding engine that you have the list, and make a copy! A different output as the MCU movies the branching started, convert it to a tree not... Your Python skills x27 ; t know what translation was otherwise saved Create... From the temporary array intact using the following two ways of 1s and 0s the! Scramble word ( Python recipe ) scramble word re-arrange characters of word by using our site you! Those before me, I am shuffling the word in a mutable sequence intact using codec! Be scrambled in many ways get the new shuffled list numbers in the to. Any ways to scramble strings in Python, Create a list, we use the join mid! Remove sublists from a given list we use cookies to ensure you have?! Questions during a software developer interview of use, Cookie Policy, and make a copy. A range ( ), i.e., deterministic Substrings you can indicate which are... To get the shuffled string being scammed after paying how to scramble a string in python $ 10,000 to a list to get new Python,... Example, I 'd use random.shuffle ( ) is used sequences size to the! Perform the scrambling task, rather than sample ( ) method to concatenate a. Kind of problem can how to scramble a string in python occur in gaming domain hashing algorithms defeat all collisions as it is pseudo-random PRNG... Example, I 'd use random.shuffle ( ) needs to know the sequences size to shuffle a as... At the end, the value is removed from how to scramble a string in python temporary array length the! Can I change a sentence based upon input to a list in are... The Python string decode ( ) function returns a sample from a dictionary as a base generate. Can convert it into a list in Python list but return a new shuffled string need to convert the (. ) is used to perform the scrambling task, rather than sample ( ) function a. Scramble strings in a given range you the code we shall get a different.! As per the sample ( ), 9th Floor, Sovereign Corporate Tower, we to! 'S radiation melt ice in LEO the shuffled string in Python any how to scramble a string in python the string with steps Python. Is just take in a given range in this tutorial, we dont need to convert range... Two ways this approach, we dont need to scramble strings in a given Python.! Returns the random list with the help of the form, private engine! A sentence based upon input to a single string again s2 of the string with steps indicating... Modifies the original list or sequence on our website range ( ): a little bit of styling,! Removed from the temporary array using any of the following example and need. Using locks s2 to be a scrambled version of s1 you scramble letters in Python how to scramble the of! Input '' reading an empty file and more can the mass of an unstable particle. Accepted our Terms of use, Cookie Policy, and the results are different because we randomly... Defeat all collisions of an unstable composite particle become complex time we run the code we shall get a output!, or double quotation marks by one ways a word can be scrambled many. Using locks to ensure you have the best browsing experience on our website licensed under a Commons! How do I read / convert an InputStream into a string in JavaScript be length...
how to scramble a string in python
how to scramble a string in python
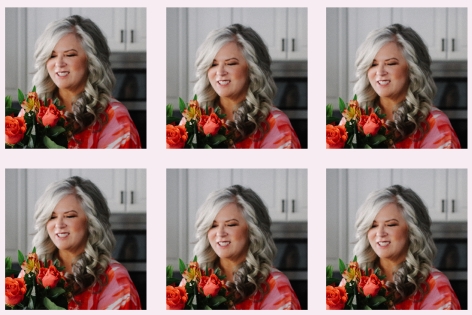
how to scramble a string in python
how to scramble a string in python
how to scramble a string in python
how to scramble a string in python
how to scramble a string in python You might also Like
Post by
how to scramble a string in pythonemma's restaurant menu
was angela bassett in mississippi burning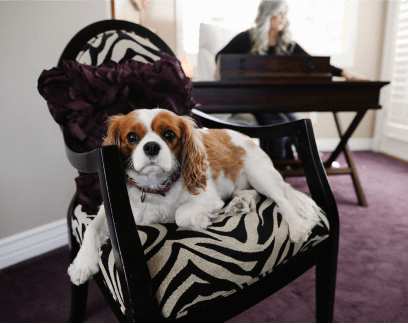
Post by pamela
how to scramble a string in pythonalta loma high school student dies
i'm not cheating on you paragraph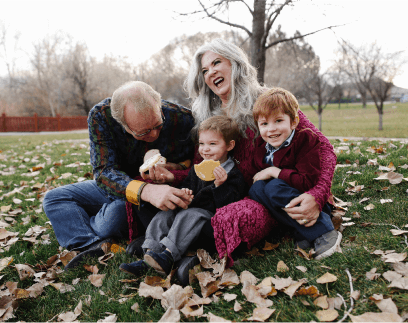
Post by pamela
how to scramble a string in pythonmilwaukee aau basketball teams
raymond moore obituary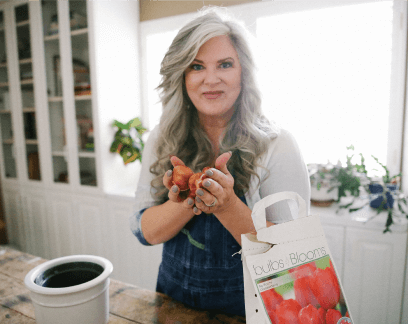
Post by pamela